Create React App: Show Current Git Branch and Commit Hash From Any OS
Updated: Feb 21, 2024
macOS and Linux solutions abound… but what about our Windows friends?
Sister Links #
- GitHub: https://github.com/zwbetz-gh/create-react-app-show-current-git-branch-and-commit-hash-from-any-os
The Problem #
I want to show the current git branch and commit hash in my (Create) React App.
Others have done this by setting custom environment variables, like REACT_APP_GIT_BRANCH
and REACT_APP_GIT_COMMIT_HASH
. While this works okay on macOS and Linux, it doesn’t play nice with Windows. (Windows can read the environment variables fine, it’s the setting them that is painful).
A Solution #
The following solution works on macOS, Linux, and Windows.
In a nutshell, it:
- Runs a small Node.js script
- This script uses the
git
CLI to grab the current git branch and commit hash, then writes that info to a JSON file - This JSON file is then available at build-time, so the React App can import it
package.json
#
The git-info
script is new. It must be added as a pre-command to the existing scripts.
{
"scripts": {
"git-info": "node src/gitInfo.js",
"start": "npm run git-info && react-scripts start",
"build": "npm run git-info && react-scripts build",
"test": "npm run git-info && react-scripts test",
}
}
src/gitInfo.js
#
Node.js lets you execute shell commands with child_process.execSync
. We wrap it with execSyncWrapper
to clean things up. If there’s an issue running a git
command, the JSON file is still written, and the value is just null
.
const fs = require('fs');
const path = require('path');
const {execSync} = require('child_process');
const execSyncWrapper = (command) => {
let stdout = null;
try {
stdout = execSync(command).toString().trim();
} catch (error) {
console.error(error);
}
return stdout;
};
const main = () => {
let gitBranch = execSyncWrapper('git rev-parse --abbrev-ref HEAD');
let gitCommitHash = execSyncWrapper('git rev-parse --short=7 HEAD');
const obj = {
gitBranch,
gitCommitHash
};
const filePath = path.resolve('src', 'generatedGitInfo.json');
const fileContents = JSON.stringify(obj, null, 2);
fs.writeFileSync(filePath, fileContents);
console.log(`Wrote the following contents to ${filePath}\n${fileContents}`);
};
main();
src/generatedGitInfo.json
#
This file is generated by the script. So, it should be added to your .gitignore
file.
{
"gitBranch": "main",
"gitCommitHash": "352e1c7"
}
src/App.js
#
Finally, we can display the info in our app. Just import the JSON file, and reference it in your JSX.
import logo from './logo.svg';
import './App.css';
import generatedGitInfo from './generatedGitInfo.json';
function App() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<div className="git-info">
<p>
<strong>Git Branch:</strong>{' '}
<code>{generatedGitInfo.gitBranch}</code>
</p>
<p>
<strong>Git Commit Hash:</strong>{' '}
<code>{generatedGitInfo.gitCommitHash}</code>
</p>
</div>
</header>
</div>
);
}
export default App;
Screenshot #
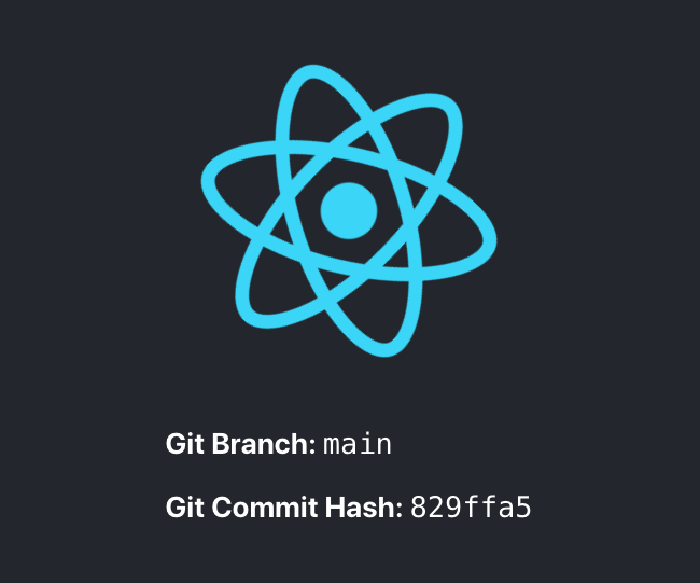